Python application development is on the rise as it is one of the most popular programming languages in the world today. And for good reasons. Its simplicity and readability make it easy to learn, even for beginners, while its versatility and power make it an ideal choice for more experienced developers. From Backend web development to data analysis, Python has a wide range of applications and is an essential tool for anyone looking to build software applications in the modern age.
In this blog, we will explore the world of Python application development, covering everything from the basics of the language to advanced techniques and best practices. Whether you are just starting out or are a seasoned developer, this blog will provide you with the knowledge you need to create powerful and efficient Python applications that meet the needs of your users. So, let's dive in and start building!
A Brief Overview of Python
Python is an interpreted, dynamically-typed, high-level programming language that is designed to be easy to read and write. It has a simple syntax that emphasizes code readability and encourages programmers to write clear and concise code. Python supports multiple programming paradigms, including object-oriented, functional, and procedural programming, making it a versatile language.
Python has a large standard library that provides developers with a wide range of tools and functions to create various applications. Additionally, Python has an extensive community that has created many third-party libraries, frameworks, and tools to make application development easier and faster.
Importance of Python in Application Development
Various industries make extensive use of Python, including data science, machine learning, frontend and backend web development game development, and scientific computing. Its popularity is due to its ease of use, quick development cycles, and wide range of applications. Python's simplicity and readability make it easy for developers to learn and write code faster than other programming languages, resulting in faster development cycles.
Python's ecosystem has a vast selection of libraries, frameworks, and tools that make it easy to create complex applications quickly. For instance, Django and Flask are popular web frameworks that allow developers to create robust web applications with minimal code. TensorFlow and PyTorch are popular machine-learning libraries that make it easy for developers to create and train machine-learning models.
Basics of Python Programming
Python is a versatile programming language that supports multiple programming paradigms, including procedural, functional, and object-oriented programming. In this section, we will explore the basics of Python programming, including variables and data types, control structures, functions and modules, and object-oriented programming.
1. Variables and Data Types
In Python, variables are used to store values, such as numbers, strings, or Boolean values. A variable can be created by assigning a value to a name using the assignment operator (=). For example, x = 10 creates a variable named x and assigns it the value 10.
Python supports various data types, including integers, floating-point numbers, strings, Boolean values, and more. The data type of a variable is determined dynamically at runtime, meaning that the data type of a variable can change based on the value assigned to it.
2. Control Structures
Control structures are used to control the flow of a program. Python supports several control structures, including conditional statements, loops, and exceptions.
Conditional statements, such as if-else statements, are used to execute code based on a condition. For example, if x > 5: print("x is greater than 5") will only execute the print statement if the value of x is greater than 5.
Loops, such as for loops and while loops are used to iterate over a sequence of values or to execute a block of code repeatedly. For example, for I in range(5): print(i) will iterate over the range of values from 0 to 4 and print each value.
Exceptions are used to handle errors that occur during program execution. For example, try: x = int(input("Enter a number: ")) except ValueError: print("Invalid input") will handle a ValueError exception if the user enters a non-numeric value.
3. Functions and Modules
Encapsulating a block of code that can be reused multiple times in a program is done through functions. To define a function, developers use the def keyword followed by the function name and any parameters.
For example, def add(x, y): return x + y defines a function named add that takes two parameters and returns their sum.
Organizing functions, classes, and variables into reusable code is done through modules. A module in Python is a file that contains code that other Python programs can import. For instance, the math module provides a range of mathematical functions like sqrt and sin, which other Python programs can use.
4. Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects and classes to represent real-world entities. Python supports OOP through the use of classes and objects.
A class is a blueprint for creating objects that have similar attributes and methods. A class is defined using the class keyword, followed by the class name and any properties or methods. For example, class Person: def __init__(self, name, age): self.name = name self. age = age defines a Person's class with a name and age property.
An object is an instance of a class that has its own unique properties and methods. An object is created by calling the class constructor and passing any required parameters. For example, person = Person("John", 30) creates a Person object with the name of "John" and an age of 30.
Tools and Frameworks for Python Application Development
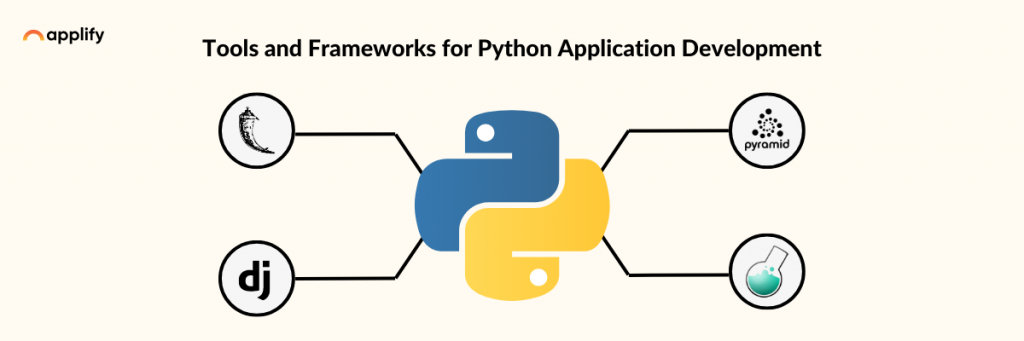
Python is a popular programming language for developing web applications, machine learning models, data analysis, and more. In this section, we will explore the various tools and frameworks available for Python application development.
1. Integrated Development Environments (IDEs)
An Integrated Development Environment (IDE) is a software application that provides a comprehensive environment for developing, testing, and debugging code. Python has several popular IDEs, including PyCharm, Visual Studio Code, and Spyder.
JetBrains developed PyCharm, a widely used IDE that offers code highlighting, code completion, code refactoring, and other features for Python development. Microsoft developed Visual Studio Code, a lightweight IDE that supports multiple programming languages, including Python. Spyder is an IDE that is specifically designed for scientific computing and data analysis in Python.
2. Code Editors
Code editors are lightweight software applications that provide a text editor for writing and editing code. While they may not provide the comprehensive features of an IDE, code editors are useful for quick prototyping and editing of Python code. Some popular code editors for Python development include Sublime Text, Atom, and Notepad++.
3. Python Libraries and Frameworks
Python has a vast ecosystem of libraries and frameworks that make it easy to develop applications for various purposes. Here are some popular Python frameworks for web application development:
- Flask
Flask is a lightweight web framework for Python that is easy to learn and use. It provides features for URL routing, template rendering, and form handling, making it a great choice for developing small to medium-sized web applications.
- Django
Django is a robust web framework for Python that follows the Model-View-Controller (MVC) architectural pattern. It provides features for URL routing, template rendering, and database modeling, making it a great choice for developing large-scale web applications.
- Pyramid
Pyramid is a flexible web framework for Python that allows developers to choose the components they need for their applications. It provides features for URL routing, template rendering, and database modeling, making it a great choice for developing web applications of any size.
- Bottle
The bottle is a minimalist web framework for Python that is easy to learn and use. It provides features for URL routing, template rendering, and database modeling, making it a great choice for developing small to medium-sized web applications.
In addition to these web frameworks, Python has several libraries for scientific computing, data analysis, machine learning, and more. Some popular Python libraries include NumPy, Pandas, Scikit-Learn, and TensorFlow.
Checkout our guide, if you want to learn about the best cross-platform development frameworks that, are popular among the developer community
Developing a Python Application
Developers can use Python, a versatile programming language, to create a wide variety of applications, ranging from simple command-line tools to complex web applications and machine-learning models. In this section, we will discuss the steps involved in developing a Python application.
1. Defining the problem
The first step in developing a Python application is to define the problem you are trying to solve. This involves understanding the problem domain, identifying the requirements, and defining the scope of the project. You should also consider factors such as user needs, performance requirements, and scalability.
2. Designing the solution
Once you have defined the problem, the next step is to design a solution. This involves breaking down the problem into smaller, more manageable tasks and designing the overall architecture of the application. You should also consider factors such as data structures, algorithms, and user interfaces.
3. Writing the code
After you have designed the solution, the next step is to write the code. This involves implementing the design using the Python programming language. You should follow best practices for coding, such as using descriptive variable names, commenting on your code, and adhering to a consistent coding style.
4. Testing the application
Once you have written the code, the next step is to test the application. This involves writing test cases and ensuring that the application behaves as expected. You should test the application in a variety of scenarios, including edge cases and unexpected inputs.
5. Debugging and Troubleshooting
After testing the application, you may encounter bugs or unexpected behavior. The final step in developing a Python application is to debug and troubleshoot the application. This involves identifying and fixing any issues that arise during testing. You should use tools such as debuggers and logging to help identify and fix bugs.
Developing a Python application involves several steps, including defining the problem, designing the solution, writing the code, testing the application, and debugging and troubleshooting. By following best practices for each step, you can develop high-quality Python applications that meet the requirements of your users.
Best Practices for Python Application Development
To ensure their Python applications are well-organized, maintainable, secure, and performant, developers should follow certain best practices. Python is a popular and powerful programming language that can be used to develop a wide range of applications, and following these practices is essential to achieve optimal results.
Developing a Python app involves defining the problem, designing the solution, writing code, testing, and debugging. Follow best practices at each step for high-quality apps that meet user requirements.
In this section, we will discuss some of the best practices for Python application development.
1. Code Organization and Project Structure
One of the most important best practices for Python application development is to have a well-organized code structure and project layout. This can help improve code maintainability, readability, and scalability. It's a good idea to follow established Python project structure conventions, such as those provided by popular tools like Cookiecutter and Flask.
2. Documentation
Another important best practice for Python application development is to have good documentation. This can help other developers understand your code and how to use your application. You should include inline documentation in your code, as well as external documentation, such as a README file, a user manual, and API documentation.
3. Testing and Continuous Integration
Testing and continuous integration are crucial for developing high-quality Python applications. You should write tests for your code to ensure that it behaves as expected and to catch any bugs early in the development process. You should also set up a continuous integration (CI) system to automate the process of building, testing, and deploying your application.
4. Security and Vulnerability Management
Security is an important consideration for any software application, and Python applications are no exception. You should follow best practices for security and vulnerability management, such as using secure coding practices, keeping your dependencies up-to-date, and conducting regular security audits.
5. Performance Optimization
Finally, you should consider performance optimization when developing Python applications. This involves identifying and fixing performance bottlenecks, using efficient algorithms and data structures, and optimizing your code for speed and memory usage. You can use profiling tools to help identify performance issues in your application.
Conclusion
Python is a powerful and versatile programming language that is continuing to grow in popularity. As more organizations embrace data-driven decision-making and machine learning, Python's capabilities in these areas are likely to become even more important. In addition, Python's ease of use and readability make it an attractive language for teaching programming to beginners. As a result, we can expect Python application development to continue to grow in popularity and importance in the coming years.